Here is how you can easily delegate SP and convert between VEST and STEEM programmatically
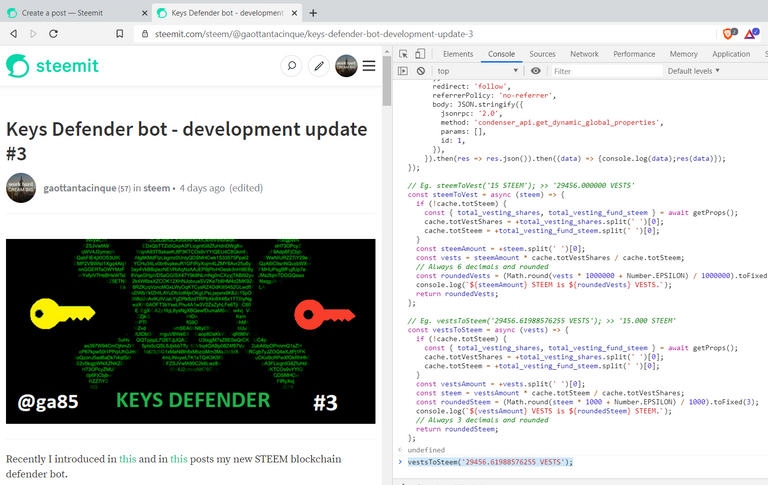
Earlier today I was trying to programmatically delegate to one of my accounts with the following (correct) code:
const client = new dsteem.Client('https://api.steemit.com');
const delegate = async (config) => {
const {
delegation, privateKey: privateActiveKey, delegator, delegatee,
} = config;
const privateKey = dsteem.PrivateKey.fromString(privateActiveKey);
const op = [
'delegate_vesting_shares',
{
delegator,
delegatee,
vesting_shares: delegation,
},
];
client.broadcast.sendOperations([op], privateKey).then(
result => console.log('Delegation done.', JSON.stringify(result)),
console.error,
);
};
const config = {
delegatee: 'bot-test',
delegator: 'marcocasario',
privateKey: '51111111111111111111111111111111111111111111111RlFx', // private active key
delegation: '29456.000000 VESTS', // Around 15 SP
};
delegate(config);
Initially though I was passing in as input STEEM instead of VEST so the script was erroring out. After reading a bit around I found out how to easily convert one into the other. Here is the JS code:
const cache = {
totVestShares: null,
totSteem: null,
};
const getProps = () => new Promise((res) => {
fetch('https://api.steemit.com', {
method: 'POST',
cache: 'no-cache',
credentials: 'omit',
headers: {
'Content-Type': 'application/json',
},
redirect: 'follow',
referrerPolicy: 'no-referrer',
body: JSON.stringify({
jsonrpc: '2.0',
method: 'condenser_api.get_dynamic_global_properties',
params: [],
id: 1,
}),
}).then(res => res.json()).then((data) => {console.log(data);res(data.result)});
});
// Usage: steemToVest('15 STEEM'); >> '29456.000000 VESTS'
const steemToVest = async (steem) => {
if (!cache.totSteem) {
const { total_vesting_shares, total_vesting_fund_steem } = await getProps();
cache.totVestShares = +total_vesting_shares.split(' ')[0];
cache.totSteem = +total_vesting_fund_steem.split(' ')[0];
}
const steemAmount = +steem.split(' ')[0];
const vests = steemAmount * cache.totVestShares / cache.totSteem;
// Always 6 decimals and rounded
const roundedVests = (Math.round(vests * 1000000 + Number.EPSILON) / 1000000).toFixed(6);
console.log(`${steemAmount} STEEM is ${roundedVests} VESTS.`);
return roundedVests;
};
// Usage: vestsToSteem('29456.61988576255 VESTS'); >> '15.000 STEEM'
const vestsToSteem = async (vests) => {
if (!cache.totSteem) {
const { total_vesting_shares, total_vesting_fund_steem } = await getProps();
cache.totVestShares = +total_vesting_shares.split(' ')[0];
cache.totSteem = +total_vesting_fund_steem.split(' ')[0];
}
const vestsAmount = +vests.split(' ')[0];
const steem = vestsAmount * cache.totSteem / cache.totVestShares;
const roundedSteem = (Math.round(steem * 1000 + Number.EPSILON) / 1000).toFixed(3);
console.log(`${vestsAmount} VESTS is ${roundedSteem} STEEM.`);
// Always 3 decimals and rounded
return roundedSteem;
};
USAGE:
This is even easier than retrieving balances of a list of Steemit accounts or calculate an account's RC programmatically as there is no need to import SteemJs or SteemdJs for the VEST <=> STEEM conversion.The script simply uses a cross domain http requests. This means that you can try this simple script in any webpage.
Step by step instructions:
STEP 1
Open your favorite browser on any site (I strongly recommend using Brave browser) and open the DevTools (Ctrl + Shift + J on Linux/Windows and Cmd + Opt + J on Mac).
STEP 2
Copy and paste my script above in the Console.
STEP 3
Press enter and there you go! You can now execute the 2 commands to convert between STEEM and VESTS.
Enjoy!! =]
NOTE:
As usual, this script is completely safe as it does not require any sort of keys to function and can be executed in any webpage!
View or trade
BEER
.Hey @gaottantacinque, here is a little bit of
BEER
from @eii for you. Enjoy it!Learn how to earn FREE BEER each day by staking.
!COFFEEA
!shop
$trdo
!BEER
Congratulations @eii, you successfuly trended the post shared by @gaottantacinque!
@gaottantacinque will receive 0.04182638 TRDO & @eii will get 0.02788425 TRDO curation in 3 Days from Post Created Date!
"Call TRDO, Your Comment Worth Something!"
To view or trade TRDO go to steem-engine.com
Join TRDO Discord Channel or Join TRDO Web Site
Congratulations @gaottantacinque, your post successfully recieved 0.04182638 TRDO from below listed TRENDO callers:
To view or trade TRDO go to steem-engine.com
Join TRDO Discord Channel or Join TRDO Web Site