[JAVA #13] [Web Automation #13] Selenium With TestNG #2 Asserting [KR]
image source: pixabay
자동화 테스트에서 가장 중요한 것은 기대결과가 예상대로 노출되었는지 확인하는 것이다.
예를 들면 글 목록이 정상적으로 노출되는지, 목록의 글 제목, 저자명, 명성, 글 내용, 저자 썸네일 등이 노출되는지를 모두 체크해야 한다.
또한 어떤 태그가 노출되지 않았는지, 무슨 이유로 노출되지 않았는지 정확하게 기록하는 것도 필요하다.
TestNG Asserting은 바로 이러한 것을 처리하기 위해 존재하며 주로 아래와 같은 asserting이 가장 많이 사용된다. (적어도 내 코드에는 자주 나타난다.)
// object가 null이 아닌지 체크, fail 발생했을 경우 리포트에 message 추가
Assert.assertNotNull(object, message);
// condition이 True인지 체크, fail 발생했을 경우 리포트에 message 추가
Assert.assertTrue(condition, message);
// Assert.assertTrue와 반대의 개념
Assert.assertFalse(condition, message);
//actual과 expected가 똑같은지 체크(타입도 체크함), fail 발생했을 경우 리포트에 message 추가
Assert.assertEquals(actual, expected, message);
👆 먼저 import org.testng.Assert.*;
라이브러리 import 후 코드는 위처럼 작성하면 된다. static 으로 import 하면 코드에 Assert.는 안적어도 된다. 👇
import static org.testng.Assert.assertEquals;
import static org.testng.Assert.assertNotNull;
import static org.testng.Assert.assertTrue;
...
...
assertNotNull(object, message);
assertTrue(condition, message);
assertFalse(condition, message);
assertEquals(actual, expected, message);
👇 아래 코드는 1)글 목록이 노출되었는지 2)비밀번호 태그를 불러왔는지 3)글쓰기 버튼이 노출되는지를 체크한다.
3번은 일부러 fail이 발생하게 체크했다.
스팀잇 페이지 dom에 글쓰기 버튼이 하나가 있는데 일부러 두개 있는지 체크하여 fail을 발생시킨다.
package com.steem.webatuo;
import static org.testng.Assert.assertEquals;
import static org.testng.Assert.assertNotNull;
import static org.testng.Assert.assertTrue;
import java.util.List;
import java.util.concurrent.TimeUnit;
import org.openqa.selenium.By;
import org.openqa.selenium.Keys;
import org.openqa.selenium.WebDriver;
import org.openqa.selenium.WebElement;
import org.openqa.selenium.chrome.ChromeDriver;
import org.testng.annotations.AfterClass;
import org.testng.annotations.BeforeClass;
import org.testng.annotations.Test;
import io.github.bonigarcia.wdm.WebDriverManager;
public class Steemit {
WebDriver driver;
@BeforeClass
public void SetUp() {
WebDriverManager.chromedriver().setup();
driver = new ChromeDriver();
driver.get("STEEMIT URL");
driver.manage().window().maximize();
driver.manage().timeouts().implicitlyWait(3, TimeUnit.SECONDS);
}
@Test
public void CASE_01_GetPosts() {
List<WebElement> list = driver.findElements(By.xpath("//div[@id='posts_list']/ul/li"));
assertTrue(!list.isEmpty(), "글 목록이 있는지 확인");
for (WebElement post : list) {
String title = post.findElement(By.xpath("descendant::h2/a")).getText();
String author = post.findElement(By.xpath("descendant::span[@class='user__name']")).getText();
System.out.println(author + "님의 글: " + title);
}
}
@Test
public void CASE_02_Login() throws InterruptedException {
// Click the login button
driver.findElement(By.linkText("로그인")).click();
// type id
driver.findElement(By.name("username")).sendKeys("june0620");
// type pw and submit
WebElement pw = driver.findElement(By.name("password"));
assertNotNull(pw, "비밀번호 태그가 노출되는지 확인");
pw.sendKeys(Password.getPw());
pw.submit();
Thread.sleep(5000);
}
@Test
public void CASE_03_Write() throws InterruptedException {
// Click the write Button
List<WebElement> writeBtns = driver.findElements(By.xpath("//a[@href='/submit.html']"));
assertEquals(writeBtns.size(), 2, "글쓰기 버튼이 노출되는지 확인");
for (WebElement writeBtn : writeBtns) {
if (writeBtn.isDisplayed()) {
writeBtn.click();
Thread.sleep(2000);
// type Text and Keys
WebElement editor = driver.findElement(By.xpath("//textarea[@name='body']"));
String keys = Keys.chord(Keys.LEFT_SHIFT, Keys.ENTER);
editor.sendKeys("hello!! world", keys, "hello!!! STEEMIT", Keys.ENTER, "안녕, 스팀잇", Keys.ENTER, "你好!似提姆");
break;
}
}
Thread.sleep(5000);
}
@AfterClass
public void tearDownClass() {
driver.quit();
}
}
실행 해 보면 아래와 같이 fail 이 발생하고 리포트에 정확하게 내가 작성한 메시지가 찍히는 것을 볼 수 있다.
만약 리포트에 한글이 깨질 경우 Eclipse가 설치된 경로에서 eclipse.ini 파일 맨 아래에 -Dfile.encoding=UTF-8
를 넣으면 해결된다. 보통 경로는 C:\Users\{사용자 컴퓨터 이름}\eclipse
이다. 👇
추가로 아래와 같은 메소드도 지원하지만 자주 다뤄보지 않아서 잘 모르겠다. 나중에 좀 친해져 보도록 하고 오늘은 이만 자즈~~아👇
assertEqualsDeep(actual, expected);
assertEqualsNoOrder(actual, expected);
assertNotEquals(actual, expected);
assertNotEqualsDeep(actual, expected);
assertSame(actual, expected);
assertNotSame(actual, expected);
assertNull(object, message);
assertThrows(throwableClass, runnable);
.
.
.
.
[Cookie 😅]
Seleniun java lib version: 3.141.59
java version: 13.0.1
@tipu curate
Upvoted 👌 (Mana: 15/25 - need recharge?)
擦 算你的还是算我的呢😂
Posted using Partiko Android
应该是算你的了 😁😀
其实他比我快12秒好像 我后来看见系统显示他时间是48秒前 我是36秒前 那个时候替普是点了5% 后来我再进去看也扣了我的 又变成点赞10%了 估计是替普系统出错了 反正多点赞到你就够了🤭
Posted using Partiko Android
?
감사합니다^^
Hi🙋
@tipu curate 2
!shop
Posted using Partiko Android
Upvoted 👌 (Mana: 10/20 - need recharge?)
晚上哈喽啊 😁
多谢萍萍无私的奉献 哈哈
我没奉献神马呀😅
Posted using Partiko Android
你好鸭,june0620!
@annepink给您叫了一份外卖!
由 @team-cn 新手村 迎着飓风 骑着村长家后院的鹿 给您送来
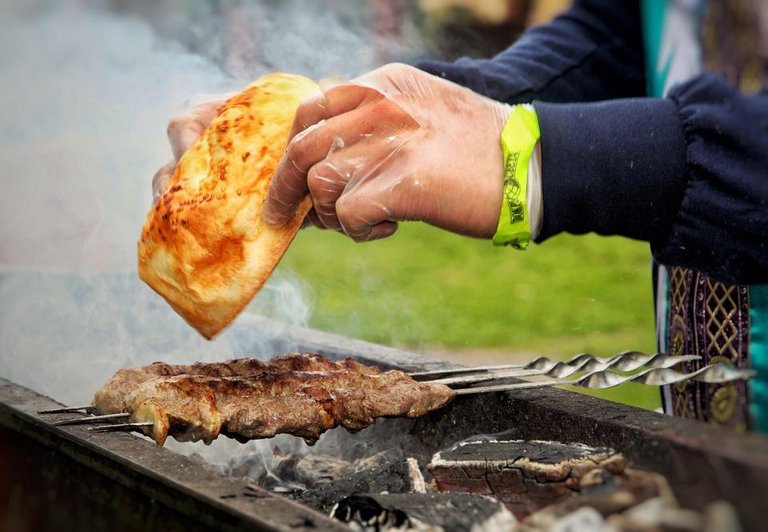
烤肉
吃饱了吗?跟我猜拳吧! 石头,剪刀,布~
如果您对我的服务满意,请不要吝啬您的点赞~
@onepagex
According to the Bible, The Book of Judas: Should You Believe It?
Watch the Video below to know the Answer...
(Sorry for sending this comment. We are not looking for our self profit, our intentions is to preach the words of God in any means possible.)
Comment what you understand of our Youtube Video to receive our full votes. We have 30,000 #SteemPower. It's our little way to Thank you, our beloved friend.
Check our Discord Chat
Join our Official Community: https://steemit.com/created/hive-182074