My second android application
Hey all,
Welcome back to my blog. Today I wanted to write about the android application which I write as a beginner. It was my longest wish to develop an android application and enjoy the usage of it. This program doesn't contain extraordinary content it is just a simple program which gets two input and performs a three variety of arithmetic calculation. Below screenshot, UI explains it clearly.
We can give two inputs in edit text fields and just press the button which your performed operations need to do.
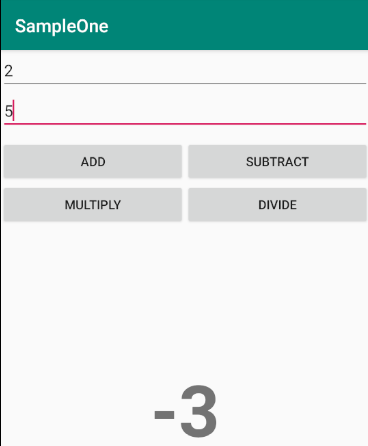
Here is the codes for the operation.
XML codes which is UI
`
<EditText
android:id="@+id/input1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter the first input"
android:inputType="number"
android:gravity="left"/>
<EditText
android:id="@+id/input2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:hint="Enter the second text"
android:inputType="number"
android:gravity="left"/>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/ButtonAdd"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="ADD"
android:layout_weight="1"
android:layout_marginTop="10dp"/>
<Button
android:id="@+id/ButtonSub"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="SUBTRACT"
android:layout_weight="1"
android:layout_marginTop="10dp"/>
</LinearLayout>
<LinearLayout
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="horizontal">
<Button
android:id="@+id/ButtonMul"
android:layout_width="100dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="MULTIPLY" />
<Button
android:id="@+id/ButtonDivide"
android:layout_width="100dp"
android:layout_weight="1"
android:layout_height="wrap_content"
android:text="DIVIDE"/>
</LinearLayout>
<TextView
android:id="@+id/result"
android:gravity="center"
android:textStyle="bold"
android:textSize="80dp"
android:layout_width="match_parent"
android:layout_height="match_parent"/>
`
While creating every Button or text view whatever it maybe we should need to name them with id name. For example, Button MUlTIPLY created id as android:id="@+id/ButtonMul"
This is because every element we created in XML has a purpose to function according to our requirement.
We need to create a new class which is giving the definition for the repeatedly using functions(helps in the reuse of codes).
I have named the class name as Arithmetic
package com.example.sampleone;
public class Arithmatic {
int add(int a, int b) {
return a + b;
}
int sub(int a, int b) {
return a - b;
}
int mul(int a, int b) {
return a * b;
}
int div(int a, int b) {
return a / b;
}
}
Here the class name is Arithmatic and the function name is add, sub, mul, div and following code explains the functions. we have to create the object in main class to access the code of class and its function. While creating the object only the required memory space is allocated in compiler. Lets see the codes inside the main_activity.java
package com.example.sampleone;
import androidx.appcompat.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
Arithmatic use = new Arithmatic();
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button buttonadd = (Button) findViewById(R.id.ButtonAdd);
Button buttonsub = (Button) findViewById(R.id.ButtonSub);
Button buttonmul = (Button) findViewById(R.id.ButtonMul);
Button buttondivide = (Button) findViewById(R.id.ButtonDivide);
buttonadd.setOnClickListener(this);
buttonsub.setOnClickListener(this);
buttonmul.setOnClickListener(this);
buttondivide.setOnClickListener(this);
}
@Override
public void onClick(View view) {
EditText firstinput = (EditText) findViewById(R.id.input1);
EditText secondinput = (EditText) findViewById(R.id.input2);
int a= Integer.parseInt(firstinput.getText().toString());
int b= Integer.parseInt(secondinput.getText().toString());
TextView resulttext = (TextView)findViewById(R.id.result);
switch (view.getId()) {
case R.id.ButtonAdd:
int z = use.add(a,b);
resulttext.setText(z + "");
break;
case R.id.ButtonSub:
int x = use.sub(a,b);
resulttext.setText(x + "");
break;
case R.id.ButtonMul:
int y = use.mul(a,b);
resulttext.setText(y + "");
break;
case R.id.ButtonDivide:
int w = use.div(a,b);
resulttext.setText(w + "");
break;
}
}
}
In this code, we referred the ID from XML code Button buttonadd = (Button) findViewById(R.id.ButtonAdd);
This code we are saying I am initializing Button named buttonadd which is the actual function UI id(XML code ) as ButtonAdd.
Arithmatic use = new Arithmatic();
This code represents object creation at the starting of the program. When compiler reads this line allocates the memory space.
And we are using a case statement in order to get the result of the clicked button and the output is printed.
If you have any doubt kindly register in comment section.
Why did an all-powerful God murder the Haitian people during the earthquake? Comment what you understand of our topic.
Check our Discord 😍🤗❤
https://discord.gg/vzHFNd6